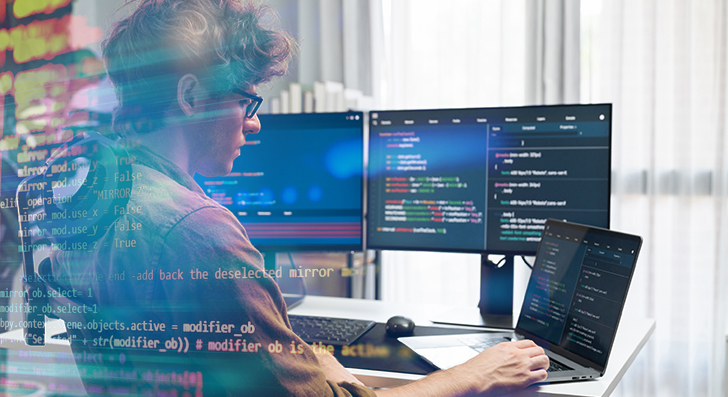
Scalability signifies your software can cope with progress—much more users, extra knowledge, and a lot more site visitors—devoid of breaking. As being a developer, developing with scalability in your mind saves time and tension afterwards. Listed here’s a clear and sensible manual to help you get started by Gustavo Woltmann.
Style and design for Scalability from the Start
Scalability just isn't anything you bolt on later—it ought to be part of your respective program from the start. A lot of programs are unsuccessful if they increase speedy mainly because the original style and design can’t deal with the additional load. As being a developer, you might want to Feel early regarding how your technique will behave stressed.
Commence by building your architecture for being adaptable. Stay away from monolithic codebases where almost everything is tightly connected. Alternatively, use modular structure or microservices. These patterns split your application into smaller, impartial sections. Every module or assistance can scale on its own devoid of influencing The entire technique.
Also, think about your database from day a person. Will it require to deal with 1,000,000 people or just a hundred? Choose the appropriate form—relational or NoSQL—based on how your details will develop. Program for sharding, indexing, and backups early, Even though you don’t need to have them still.
Another essential level is in order to avoid hardcoding assumptions. Don’t publish code that only functions below existing problems. Contemplate what would transpire In the event your person foundation doubled tomorrow. Would your application crash? Would the database decelerate?
Use style and design styles that guidance scaling, like concept queues or celebration-pushed programs. These support your app manage additional requests devoid of finding overloaded.
Any time you Establish with scalability in your mind, you are not just getting ready for success—you're decreasing potential head aches. A nicely-planned procedure is less complicated to take care of, adapt, and increase. It’s superior to get ready early than to rebuild later on.
Use the correct Databases
Selecting the correct databases is often a essential Element of making scalable apps. Not all databases are developed exactly the same, and using the Incorrect you can sluggish you down or perhaps cause failures as your application grows.
Commence by understanding your details. Can it be highly structured, like rows inside of a table? If Sure, a relational database like PostgreSQL or MySQL is a good in good shape. They are strong with associations, transactions, and consistency. In addition they assistance scaling procedures like examine replicas, indexing, and partitioning to deal with extra targeted traffic and knowledge.
In the event your info is more versatile—like consumer exercise logs, solution catalogs, or files—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at handling massive volumes of unstructured or semi-structured data and may scale horizontally extra quickly.
Also, contemplate your examine and create designs. Are you presently undertaking many reads with fewer writes? Use caching and browse replicas. Are you dealing with a heavy publish load? Take a look at databases that will cope with high publish throughput, or simply event-primarily based facts storage systems like Apache Kafka (for momentary details streams).
It’s also intelligent to Feel forward. You might not have to have Sophisticated scaling functions now, but picking a databases that supports them usually means you received’t need to have to modify afterwards.
Use indexing to hurry up queries. Stay clear of unnecessary joins. Normalize or denormalize your information according to your accessibility designs. And often keep an eye on databases performance as you grow.
In short, the proper database depends on your app’s structure, velocity requires, And exactly how you hope it to develop. Consider time to pick wisely—it’ll help you save loads of hassle afterwards.
Enhance Code and Queries
Quickly code is key to scalability. As your app grows, every little delay provides up. Inadequately prepared code or unoptimized queries can slow down overall performance and overload your method. That’s why it’s important to Establish successful logic from the beginning.
Begin by writing thoroughly clean, simple code. Stay clear of repeating logic and take away anything at all pointless. Don’t pick the most intricate Remedy if a simple just one performs. Keep your functions shorter, centered, and easy to check. Use profiling resources to find bottlenecks—destinations in which your code takes far too extended to operate or employs excessive memory.
Subsequent, check out your database queries. These normally sluggish matters down a lot more than the code itself. Be sure Every question only asks for the data you really require. Stay clear of Pick *, which fetches all the things, and as an alternative find certain fields. Use indexes to hurry up lookups. And steer clear of executing too many joins, Specially throughout big tables.
When you recognize a similar info staying asked for repeatedly, use caching. Retail outlet the results briefly working with tools like Redis or Memcached which means you don’t should repeat expensive operations.
Also, batch your databases functions whenever you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and makes your app far more successful.
Make sure to test with big datasets. Code and queries that operate high-quality with 100 information may possibly crash if they have to take care of one million.
In short, scalable apps are quick apps. Keep your code tight, your queries lean, and use caching when necessary. These methods enable your application remain easy and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your app grows, it's to deal with a lot more consumers and a lot more website traffic. If anything goes by a person server, it will eventually rapidly become a bottleneck. That’s where load balancing and caching come in. Both of these applications enable maintain your app quickly, stable, and scalable.
Load balancing spreads incoming visitors throughout various servers. In place of one server accomplishing many of the do the job, the load balancer routes people to diverse servers determined by availability. This implies no single server gets overloaded. If a person server goes down, the load balancer can send out visitors to the Other individuals. Tools like Nginx, HAProxy, or cloud-based alternatives from AWS and Google Cloud make this straightforward to build.
Caching is about storing knowledge temporarily so it might be reused speedily. When end users request a similar data once more—like an item website page or even a profile—you don’t need to fetch it with the databases each and every time. You can provide it from your cache.
There's two widespread types of caching:
one. Server-side caching (like Redis or Memcached) outlets information in memory for speedy accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) suppliers static information near the consumer.
Caching cuts down database load, increases speed, and would make your app far more efficient.
Use caching for things which don’t change typically. And always be sure your cache is current when facts does alter.
Briefly, load balancing and caching are easy but highly effective tools. Collectively, they assist your app take care of extra consumers, keep speedy, and Recuperate from challenges. If you propose to develop, you need the two.
Use Cloud and Container Instruments
To build scalable programs, you require applications that let your app expand quickly. That’s where by cloud platforms and containers come in. They give you versatility, lessen set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Net Expert services (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and services as you'll need them. You don’t must get components or guess long run ability. When targeted visitors improves, you can add much more sources with just a few clicks or routinely working with car-scaling. When website traffic drops, you may scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and protection instruments. It is possible to target constructing your app as opposed to handling infrastructure.
Containers are another key Software. A container offers your application and anything it should run—code, libraries, settings—into a person device. This makes it simple to maneuver your app in between environments, from your notebook on the cloud, without having surprises. Docker is the most popular Software for this.
Whenever your app takes advantage of multiple containers, applications like Kubernetes allow you to control them. Kubernetes handles deployment, scaling, and Restoration. If one particular element of your application crashes, it restarts it instantly.
Containers also make it very easy to independent aspects of your app into services. You may update or scale elements independently, which is great for performance and trustworthiness.
In a nutshell, utilizing cloud and container instruments indicates you could scale quickly, deploy conveniently, and Recuperate immediately when difficulties materialize. If you need your application to develop devoid of limits, start off using these equipment early. They help you save time, decrease possibility, and make it easier to stay focused on making, not correcting.
Check Anything
In the event you don’t keep an eye on your software, you received’t know when issues go Erroneous. Checking assists you see how your application is accomplishing, spot concerns early, and make superior conclusions as your app grows. It’s a important Element of developing scalable techniques.
Start out by monitoring basic metrics like CPU usage, memory, disk Room, and reaction time. These inform you how your servers and products and services are performing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this details.
Don’t just monitor your servers—keep track of your app as well. Keep watch over just how long it requires for people to load web pages, how often errors occur, and exactly where they take place. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Setup alerts for essential issues. As an example, Should your response time goes above a limit or simply a company goes down, you should get notified immediately. This allows you take care of difficulties rapid, generally right before buyers even detect.
Monitoring can also be useful after you make variations. When you deploy a whole new characteristic and see a spike in glitches or slowdowns, it is possible to roll it back before it will cause true harm.
As your application grows, website traffic and info increase. Devoid of monitoring, you’ll pass up indications of difficulty right until it’s much too late. But with the best resources set up, you remain on top of things.
In brief, checking aids you keep the app responsible and scalable. It’s not almost recognizing failures—it’s about knowledge your method and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t get more info only for big corporations. Even little applications need a powerful Basis. By creating cautiously, optimizing correctly, and utilizing the proper applications, it is possible to build apps that improve smoothly with no breaking under pressure. Commence smaller, Believe massive, and Establish wise.